스프링 부트의 꽃은 DI라고 합니다.
DI가 무엇이길래?
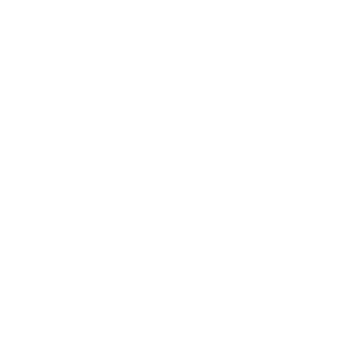
DI는 의존성 주입입니다.
의존성을 주입을 하는 이유가 뭘까요?
public class BugService {
public void bountLeg() {
BugRepository bug = new Fly();
bug.legCount();
}
}
// BugService가 Fly class에 의존된다.
위 처럼 new를 통해 직접 객체를 생성하면 의존되어 버린다는 문제점이 있습니다.
따라서 다른 객체를 사용하고 싶어도 코드를 수정하기 전까지 사용할 수 없다는 문제가 생깁니다.
따라서 객체의 밖에서 객체를 넣어주는 방법을 사용하는데 이를 의존성 주입이라고 합니다.
public class BugService {
private final BugRepository bugRepository;
public BugRepository(BugRepository bugRepository) {
this.bugRepository = bugRepository;
}
public void countLeg() {
bugRepository.legCount();
}
}
의존성은 3가지 방법으로 @Autowired라는 어노테이션을 통해 주입할 수 있습니다.
1. 생성자 주입
생성자 호출 시점에 1회 호출되는 것을 보장합니다.
필수적으로 사용하는 매개변수 없이는 인스턴스를 만들수 없어서 객체의 주입이 필요한 경우를 강제하기 위해 사용합니다. 또한 생성자를 주입함으로써 final로 불변성을 선언 할 수 있습니다.
@Controller
class Controller {
private final Repository repository;
@Autowired
public Condtroller(Repository repository) {
this.repository = repository;
}
}
// controller 와 repository 사이 의존성 연결
2. 수정자(setter) 주입
우선 객체를 생성하고 그 후에 인자를 사용하는 객체를 찾거나 만들게 됩니다.
그 이후에 주입하려는 빈 객체의 수정자를 호출해서 주입합니다. 주입받는 객체가 변경될 가능성이 있는 경우에 사용합니다.
하지만, 무분별하게 setter를 사용하게 될 경우 이를 다르게 사용할 수 있기 때문에 주의해서 사용해야 합니다.
@Controller
class Controller{
private Repository repository;
@Autowired
public void setRepository(Repository repository){
this.repository = repository;
}
}
3. 필드 주입
수정자 주입 방법과 동일하게 먼저 빈을 생성한 후에 어노테이션이 붙은 피드에 해당하는 빈을 찾아서 주입하는 방법입니다.
@Controller
class Controller{
@Autowired private Repository repository;
}
만약 주입받는 레퍼런스의 구현체가 여러개 일 경우, 어떤 빈을 주입받아야 할지 알수 없는 문제가 발생합니다.
@Service
public class BugService {
private BugRepository bugRepository;
public BugService(BugRepository bugrepository) {
this.bugRepository = bugRepository;
}
public void countLeg() {
bugRepository.legCount();
}
}
Could not autowire. There is more than one bean of 'BugRepository'type
이 경우 주입 받을 클래스에 가서 @Primary 어노테이션을 달아줍니다.
'기술 스텍 > Spring' 카테고리의 다른 글
IntelliJ 단축키(mac) (0) | 2024.11.28 |
---|---|
JPA에서 IDENTITY상태일때, persist할 때 DBMS에 반영되는가? (0) | 2024.06.17 |
Spring Cloud (0) | 2023.10.11 |